While developing an app you must have come with a usecase to select some portions of the text maybe to copy it or any other purpose.You might have wondered how to achieve this functionality if you are developing your application using a cross-platform framework like flutter.
For this usecase, you must use the SelectableText widget in Flutter which is used to create selectable texts which you can copy or select in flutter.
So let’s discuss how to use the SelectableText widget to handle text selection in Flutter.
What is SelectableText Widget in flutter?
SelectableText widget displays a string of text with a single style.This widget is useful in cases where you required you need to select or copy a particular part or entire text in flutter or in other words makes the text in your app selectable for the user.Also,the string inside the SelectableText might break across multiple lines or might all be displayed on the same line depending on the layout constraints which is also an added advantage.
How to use the SelectableText Widget in Flutter?
Using SelectableText is similar to using Text Widget in flutter,only difference is the functionality this widget provides and its parameters.
Lets first take a looks at its constructor to understand the parameters of this widget.
Constructor
SelectableText(
String data,
{Key? key,
FocusNode? focusNode,
TextStyle? style,
StrutStyle? strutStyle,
TextAlign? textAlign,
TextDirection? textDirection,
double? textScaleFactor,
bool showCursor = false,
bool autofocus = false,
ToolbarOptions? toolbarOptions,
int? minLines,
int? maxLines,
DragStartBehavior dragStartBehavior = DragStartBehavior.start,
bool enableInteractiveSelection = true,
TextSelectionControls? selectionControls,
GestureTapCallback? onTap, ScrollPhysics? scrollPhysics,
String? semanticsLabel,
TextHeightBehavior? textHeightBehavior,
TextWidthBasis? textWidthBasis,
SelectionChangedCallback? onSelectionChanged})
Properties
- autofocus: Whether this text field should focus itself if nothing else is already focused.
- data: The text to display.
- key: Controls how one widget replaces another widget in the tree.
- onSelectionChanged: Called when the user changes the selection of text (including the cursor location.
- enableInteractiveSelection: Whether to enable user interface affordances for changing the text selection.
- maxLines: The maximum number of lines to show at one time, wrapping if necessary. If this is 1 (the default), the text will not wrap, but will scroll horizontally instead.
- minLines: The minimum number of lines to occupy when the content spans fewer lines. This can be used in combination with maxLines for a varying set of behaviors.
- onTap: Called when the user taps on this selectable text. The selectable text builds a GestureDetector to handle input events like a tap.
- selectionControls: Optional delegate for building the text selection handles and toolbar.
- style: The style to use for the text. If null, defaults DefaultTextStyle of context.
- dragStartBehavior: Determines the way that drag start behavior is handled. Setting this to DragStartBehavior.start will make drag animation smoother and setting it to DragStartBehavior.down will make drag behavior feel slightly more reactive.
- showCursor: Whether to show the cursor. The cursor refers to the blinking caret when the EditableText is focused.
- scorllPhysics: The ScrollPhysics to use when vertically scrolling the input. If not specified, it will behave according to the current platform.
Implementation
Lets see how to use SelectableText widget in action.The following code is use to implement it.
SelectableText(
"This is a selectable Text.",
style: TextStyle(fontSize: 18),
)
The following code makes the text selectable instead of Text widget which doesn’t make a text selectable or has any copyable features.
But how can we make RichText widget text selectable as well.This can be achieved by using `SelectableText.rich()` widget. Let’s see how
SelectableText.rich(
TextSpan(
style: TextStyle(fontSize: 20),
children: [
TextSpan(text:"Lets,"),
TextSpan(text:"make this text selectable and copyable text.")
]
)
)
You can use `SelectableText.rich()` widget to add RichText widget and make it selectable and copyable for the user.
Complete Code to handle text selection in flutter
import 'package:flutter/material.dart';
void main(){
runApp(MyApp());
}
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Home(),
);
}
}
class Home extends StatefulWidget{
@override
_HomeState createState() => _HomeState();
}
class _HomeState extends State<Home> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Selectable Text Flutter"),
backgroundColor: Colors.redAccent,
),
body: Container(
padding: EdgeInsets.all(20),
alignment: Alignment.center,
child: Column(
children: [
SelectableText(
"This is a selectable Text.",
style: TextStyle(fontSize: 18),
),
SelectableText.rich(
TextSpan(
style: TextStyle(fontSize: 20),
children: [
TextSpan(text:"Lets,"),
TextSpan(text:"make this text selectable and copyable text.")
]
)
)
],
),
)
);
}
}
Output
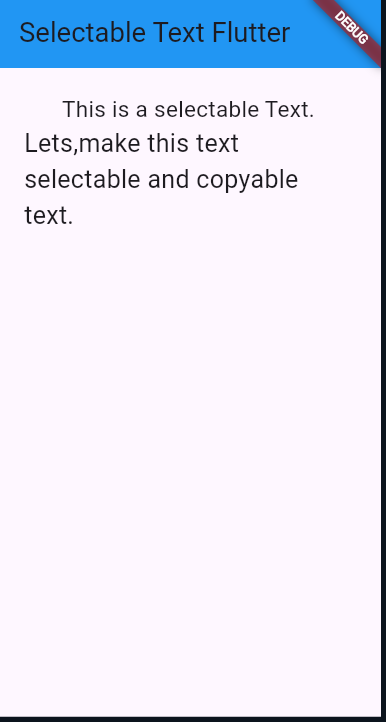
Conclusion
We learn how to handle text selection in flutter by using SelectableText
to create text which can be selectable and copyable by the user.This helps improve user experience of the user.We also learned how to use SelectableText.rich()
to add Selectable RichText
Widget which can be selected and copyable by the user.
Identifying when you need to use SelectableText
is also important.Use Text
widget when you need to display some text to the user.If you want to add something like email, or quotes that can be copyable and selectable then SelectableText
would be the widget you should consider using.
Wanna Level up Your Flutter game? Then check out our ebook The Complete Guide to Flutter Developement where we teach you how to build production grade cross platform apps from scratch.Do check it out to completely Master Flutter framework from basic to advanced level.
Also read