Hyperlink is a digital reference to data that the user can follow or be guided to by clicking or tapping. In simple words it’s a link that redirects you to a website through a url. It also can be such that by clicking a text which is underlined redirects you to a website.
You can create a hyperlink widget in Flutter. We will discuss some ways how to achieve it in this blog. We will also discuss ways on how to make an icon clickable as well. So let’s begin.
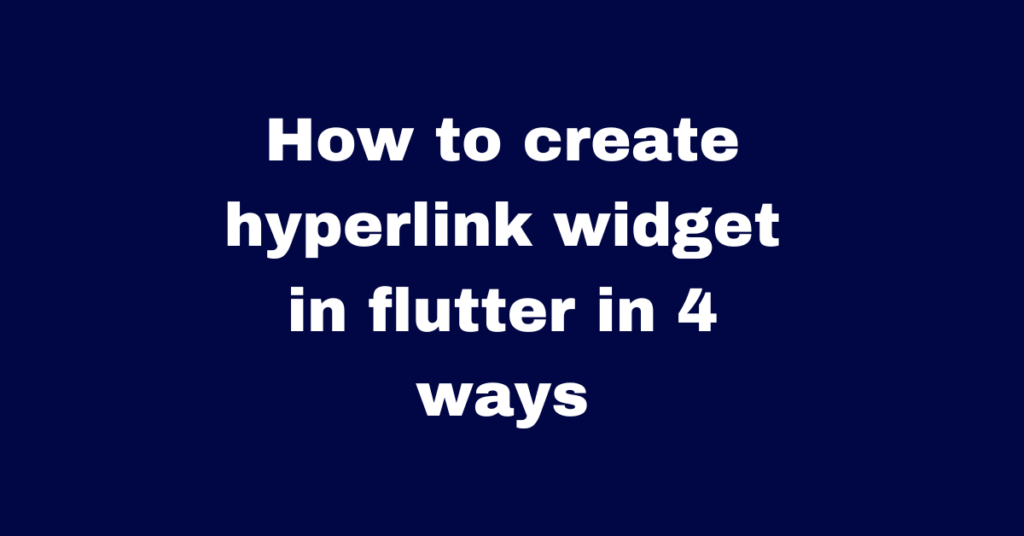
How to create a hyperlink widget in flutter?
One way to create a hyperlink widget in Flutter is by using a whole widget like InkWell,TextButton, or GestureDetector widget and using url_laucher in the onTap callback to launch a url. Since for Flutter, we don’t have an in-build widget to launch URL we use url_launcher.
First, install url_launcher
as a dependency in flutter in package.json
file.
dependencies:
url_launcher: ^6.2.5
Then, run the following command to activate the plugin
flutter pub get
get the url_launcher plugin from here:
Now let’s use a widget like GestureDetector to wrap another Text widget and launch a URL using onTap callback. Here’s the following code to achieve the desired functionality.
GestureDetector(
onTap:_launchURL(),
child: Text("Launch flutter website",style: TextStyle(
color: Colors.blue,
fontSize: 30,
fontWeight: FontWeight.bold
)
_launchURL()
is basically the function used to launch a URL. It also validates the URL by checking its valid or not if yes it launches the URL else it throws an exception.
_launchURL() async {
Uri _url = Uri.parse('https://flutter.dev');
if (await launchUrl(_url)) {
await launchUrl(_url);
} else {
throw 'Could not launch $_url';
}
}
We learned how to make a Flutter widget clickable to launch a URL, in other words how to create a hyperlink widget in Flutter. Now let’s explore some other ways as well to go about it.
How to create a text link in Flutter?
In some cases, you don’t want the whole text to be a link. You need a text within a paragraph to be a link. To achieve this functionality you would require to use a widget called RichText a combination of TextSpans. We will discuss ways how to achieve both scenarios.
Let’s first learn how to make a whole text to be a link its easy you can either wrap a Text
widget with Inkwell or GestureDetector and use OnTap callback to launch a URL as we usually do. Here’s the following code to achieve as discussed.
InkWell(
onTap: ()async{
var url = "https://flutter.dev/";
if(await canLaunch(url)){
await launch(url);
}else{
throw 'error launching $url';
}
},
child: const Text(
'Visit flutter',
style: TextStyle(
fontSize: 30,
color: Colors.blue,
decoration: TextDecoration.underline,
),
),
),
This is how we make a whole text a link using InkWell
and url_launcher
.Now let’s discuss how can we make text within a paragraph a hyperlink.
To achieve this we use RichText and TextSpan widgets in Flutter where we get granular control on each text inside a paragraph or a sentence. We can also change the style of each word or sentence which is out of the scope of this tutorial. Here’s the code to achieve the discussed functionality.
RichText(
text: TextSpan(
children: <TextSpan>[
const TextSpan(
text: 'This way we can add ',
style: TextStyle(color: Colors.black87),
),
TextSpan(
text: 'a link',
style: const TextStyle(
color: Colors.blue,
decoration: TextDecoration.underline,
),
recognizer: TapGestureRecognizer()
..onTap = () => launchUrlString('https://www.fluttercurious.com'),
),
const TextSpan(
text: ' within paragraph',
style: TextStyle(color: Colors.black87),
)
],
),
);
TextSpan
‘s recognizer property helps the user to interact with the text which launches the desired URL that we have given. Hence this is how we can add text as a link within a paragraph.
Create hyperlinks with flutter_linkify
flutter_linky is a flutter package that is used for dynamic text linking which means it turns links inside a text to tappable hyperlinks automatically.
All we need to do is wrap our Text
in the Linkify widget. The only disadvantage of this widget according to me is we can’t style the hyperlink text differently. All we can do is remove https// to make it sound less technical. Here’s the code to achieve this feat.
Linkify(
text: 'This way we can embed a link into a paragraph.\n'
'We can\'t disguise the link: https://www.flutterdev.com',
textAlign: TextAlign.center,
onOpen: (LinkableElement link) async {
await launchUrlString(link.url);
},
),
How to create a hyperlink button in Flutter?
You would like to make your button a hyperlink, that is by clicking a button you wanna redirect the user to a particular link or a website in general. You can achieve the following by using the onTap functionality of the button properties such as ElevatedButton, OutlinedButton, or any material button. Let’s write some code to see how to achieve it.
ElevatedButton(
onPressed: () async {
if (await canLaunch(url)) {
await launch(url);
} else {
// Handle case where url cannot be launched
throw 'error launching $url';
}
},
child: Text(text),
);
Here, we used ElevatedButton
then we check whether the URL can be launched or not. If yes we launch it if not we throw an exception when it can’t be launched.
How to create a hyperlink icon in Flutter?
You can also make your icons clickable or make a hyperlink icon. It’s easy you just have to use IconButton
and make use of the onPressed
property. Here’s the code you can use to achieve the following functionality.
IconButton(
icon: Icon(Icons.ac_unit,),
onPressed: () async {
const url = 'https://github.com/himanshusharma89';
if (await canLaunch(url)) {
await launch(url);
} else {
throw 'Could not launch $url';
}
}
)
Hence by the combination of IconButton and URL launcher, you can make the hyperlink icon or you can make the icon clickable.
Conclusion
In this article, we learned how to create a hyperlink widget in Flutter in 4 different ways. You can use this knowledge to make any widget clickable or to make a hyperlink widget.
Hyperlinks are an integral part of any app or website. If you need dynamic linking which is especially useful for chat messaging and all then use flutter_linkify which automatically converts text into tappable links.
If the text is already known at compile time then using RichText
or TextButton
will be suitable for the job. Also,url_launcher is a valuable package in the Flutter ecosystem for launch URLs.
Wanna Level up Your Flutter game? Then check out our ebook The Complete Guide to Flutter Developement where we teach you how to build production grade cross platform apps from scratch.Do check it out to completely Master Flutter framework from basic to advanced level.